resize事件只能加在window对象上,并不能监听具体某个DOM元素。
MutationObserver
监听DOM变动1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23function observeCHeader() {
var url = window.location.href;
var MutationObserver = window.MutationObserver || window.WebKitMutationObserver || window.MozMutationObserver;
var observer = new MutationObserver(function() {
const cheaderId = url.split('cheaderId=')[1];
if (cheaderId) {
const id = cheaderId.split('#')[0];
const dom = document.getElementById(id);
if(dom) {
dom.classList.add('active');
}
}
});
observer.observe(document.querySelector('#header_nav'), {
childList: true,
attributes: true,
characterData: true,
subtree: true,
});
}
借助window 与 getBoundingClientRect
监听点击事件,点击之后打开。
1 | // 点击出现弹出框,此处最好使用mousedown而非click |
监听鼠标离开DOM
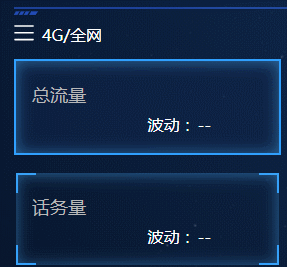
- 点击按钮,出现SiderBar(一个div)
- 鼠标在SiderBar(div)做操作。
- 当鼠标离开SiderBar(div)时,SiderBar应该消失。
1 | class SideBar extends React.Component { |